You can do a lot of things with bash scripts. Performing simple arithmetic operations with the variables is one of them.
The syntax for arithmetic operations in the bash shell is this:
$((arithmetic_operation))
Let’s say you have to calculate the sum of two variables. You do it like this:
sum=$(($num1 + $num2))
There is no restriction on the use of white space inside the (()). You can use $(( $num1+ $num2))
, $(( $num1+ $num2 ))
or $(( $num1+ $num2 ))
. It all will work the same.
Before I discuss it in detail with examples, let me share the arithmetic operators it supports.
Basic arithmetic operators in Bash
Here’s a list of the arithmetic operators in the Bash shell.
Operator | Description |
---|---|
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Integer division (without decimal) |
% | Modulus division (only remainder) |
** | Exponentiation (a to the power b) |
đźš§
Bash does not support floating points (decimals). You’ll have to use other commands like bc
to deal with them.
Addition and subtraction in bash
Let’s see it by writing a script that takes two numbers from the user and then prints their sum and subtraction.
#!/bin/bash read -p "Enter first number: " num1
read -p "Enter second number: " num2 sum=$(($num1+$num2))
sub=$(($num1-$num2))
echo "The summation of $num1 and $num2 is $sum"
echo "The substraction of $num2 from $num1 is $sub"
I believe you are familiar with using the read command to accept user input in bash from the previous chapter.
You should focus on these two lines:
sum=$(($num1+$num2))
sub=$(($num1-$num2))
Save this script as sum.sh
and run it. Give it some inputs and check the result.
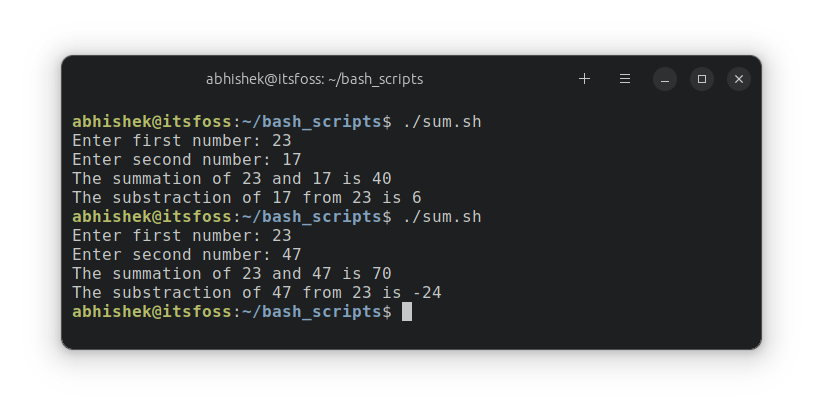
Multiplication in bash
Let’s move to multiplication now.
Here’s a sample script that converts kilometers into meters (and troubles US readers :D). For reference, 1 kilometer is equal to 1000 meters.
#!/bin/bash read -p "Enter distance in kilometers: " km
meters=$(($km*1000)) echo "$km KM equals to $meters meters"
Save the script as multi.sh
, give it execute permission and run it. Here’s a sample output:
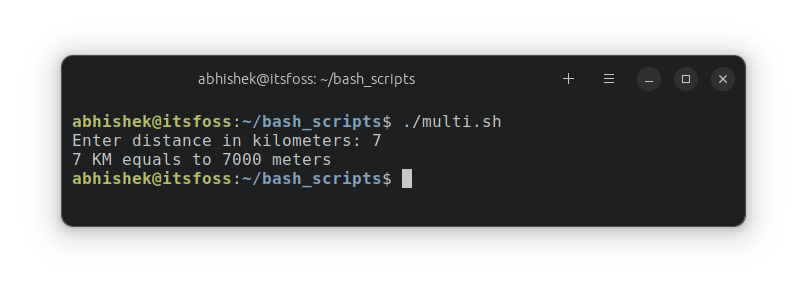
Looks good, no? Let’s move on to division.
Division in bash scripts
Let’s see division with a very simple script:
#!/bin/bash num1=50
num2=5 result=$(($num1/$num2)) echo "The result is $result"
You can easily guess the result:
The result is 10
That’s fine. But let’s change the numbers and try to divide 50 by 6. Here’s what it shows as result:
The result is 8
But that’s not correct. The correct answer should be 8.33333.
That’s because bash only deals with integers by default. You need additional CLI tools to handle floating points (decimals).
The most popular tool is bc which is quite a powerful calculator language to deal with mathematical operations. However, you don’t need to go into detail for now.
You have to ‘echo’ the arithmetic operation to bc via pipe:
echo "$num1/$num2" | bc -l
So, the previous script is modified into:
#!/bin/bash num1=50
num2=6 result=$(echo "$num1/$num2" | bc -l) echo "The result is $result"
And now you get the result:
The result is 8.33333333333333333333
Notice the result=$(echo "$num1/$num2" | bc -l)
, it now uses the command substitution that you saw in chapter 2 of this series.
The -l
option loads standard math library. By default, bc will go up to 20 decimal points. You can change the scale to something smaller in this way:
result=$(echo "scale=3; $num1/$num2" | bc -l)
Let’s see some more examples of floating points in bash.
Handling floating points in bash scripts
Let’s modify the sum.sh
script to handle floating points.
#!/bin/bash read -p "Enter first number: " num1
read -p "Enter second number: " num2 sum=$( echo "$num1+$num2" | bc -l)
sub=$( echo "scale=2; $num1-$num2" | bc -l)
echo "The summation of $num1 and $num2 is $sum"
echo "The substraction of $num2 from $num1 is $sub"
Try running it now and see if handles floating points properly or not:
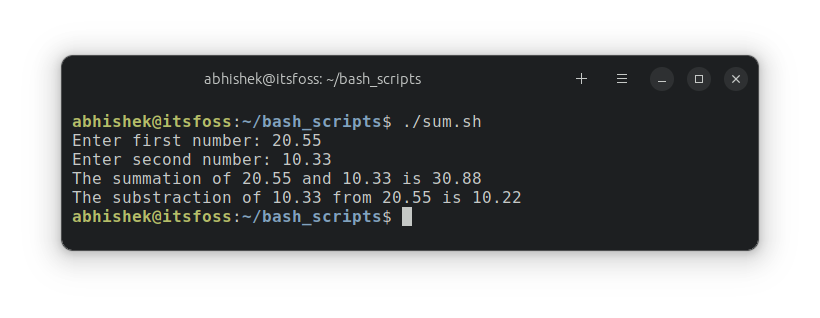
🏋️🤸 Exercise time
Time to do some maths and bash exercises together.
Exercise 1: Create a script that accepts input in GB and outputs its equivalent value in MB and KB.
Exercise 2: Write a script that takes two arguments and outputs the result in exponential format.
So, if you enter 2 and 3, the output will be 8, which is 2 to the power 3.
Hint: Use the exponentiation operator **
Exercise 3: Write a script that converts Centigrade to Fahrenheit.
Hint: Use the formula F = C x (9/5) + 32. You’ll have to use bc
command here.
You can discuss the exercises and their solution in the community.
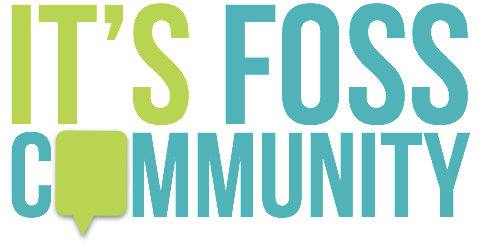
In the next chapter, you’ll learn about arrays in Bash. Stay tuned.