In the first part of the Bash Basics Series, I briefly mentioned variables. It is time to take a detailed look at them in this chapter.
If you have ever done any kind of coding, you must be familiar with the term ‘variable’.
If not, think of a variable as a box that holds up information, and this information can be changed over time.
Let’s see about using them.
Using variables in Bash shell
Open a terminal and use initialize a variable with a random number 4:
var=4
So now you have a variable named var
and its value is 4
. Want to verify it? Access the value of a variable by adding $ before the variable name. It’s called parameter expansion.
[email protected]:~$ echo The value of var is $var
The value of var is 4
🚧
There must NOT be a space before or after =
during variable initialization.
If you want, you can change the value to something else:
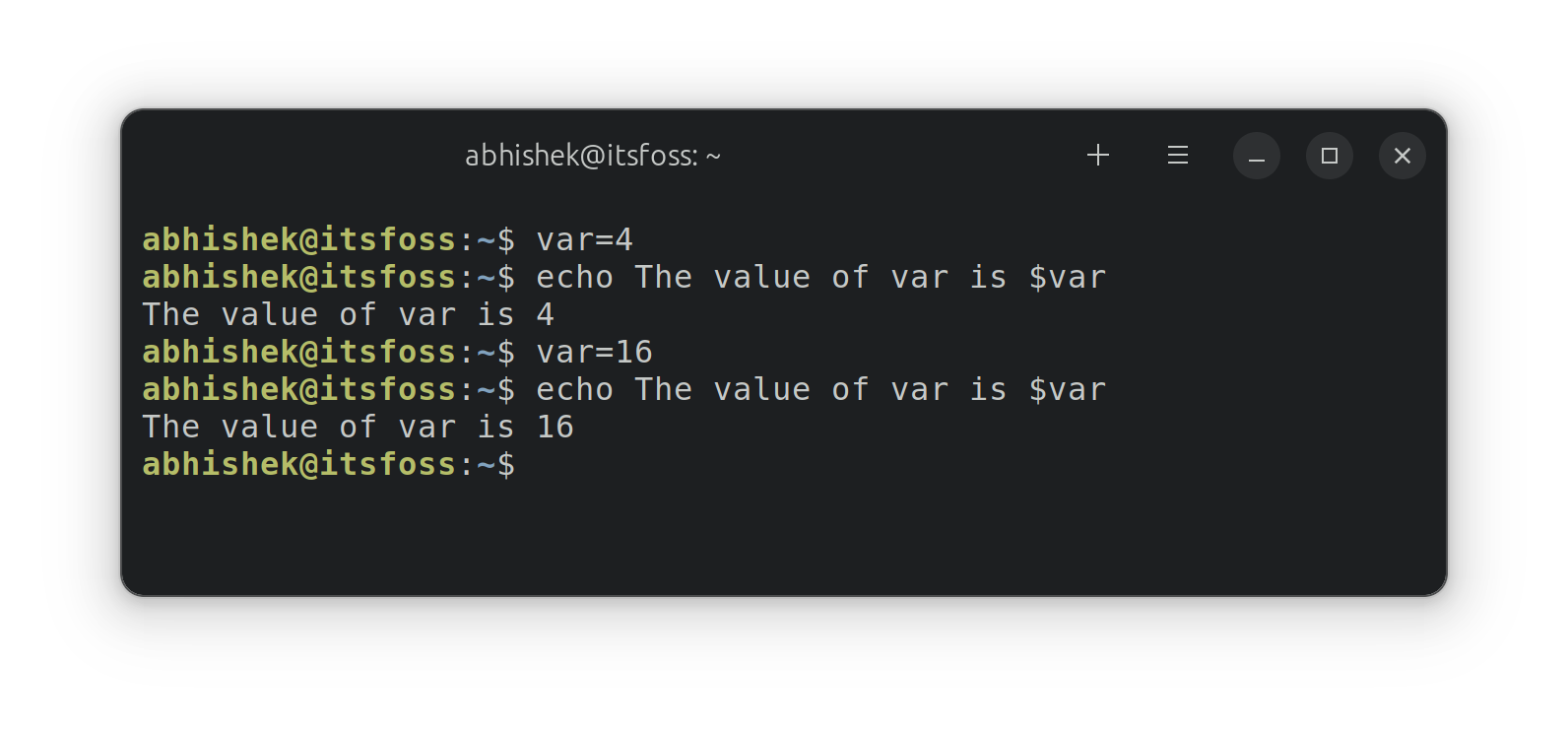
In Bash shell, a variable can be a number, character, or string (of characters including spaces).
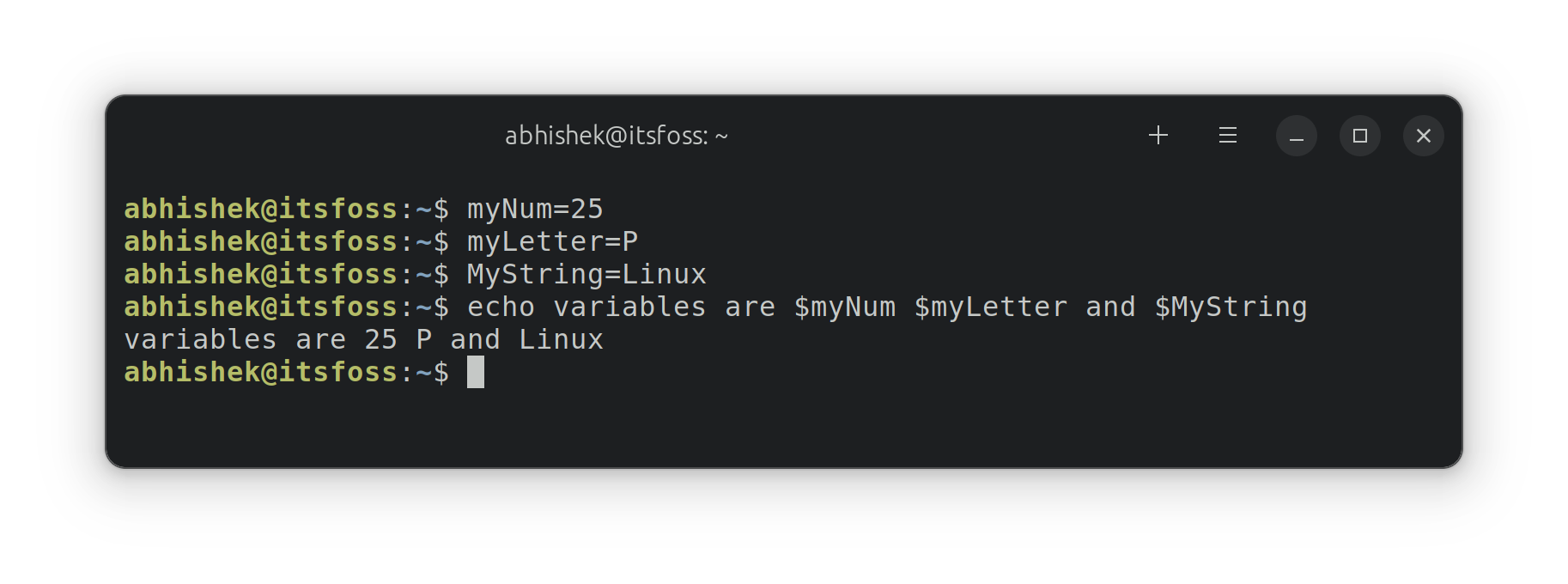
💡
Like other things in Linux, the variable names are also case-sensitive. They can consist of letters, numbers and the underscore “_”.
Using variables in Bash scripts
Did you notice that I didn’t run a shell script to show the variable examples? You can do a lot of things in the shell directly. When you close the terminal, those variables you created will no longer exist.
However, your distro usually adds global variables so that they can be accessed across all of your scripts and shells.
Let’s write some scripts again. You should have the script directory created earlier but this command will take care of that in either case:
mkdir -p bash_scripts && cd bash_scripts
Basically, it will create bash_scripts
directory if it doesn’t exist already and then switch to that directory.
Here. let’s create a new script named knock.sh
with the following text.
#!/bin/bash echo knock, knock
echo "Who's there?"
echo "It's me, $USER"
Change the file permission and run the script. You learned it in the previous chapter.
Here’s what it produced for me:
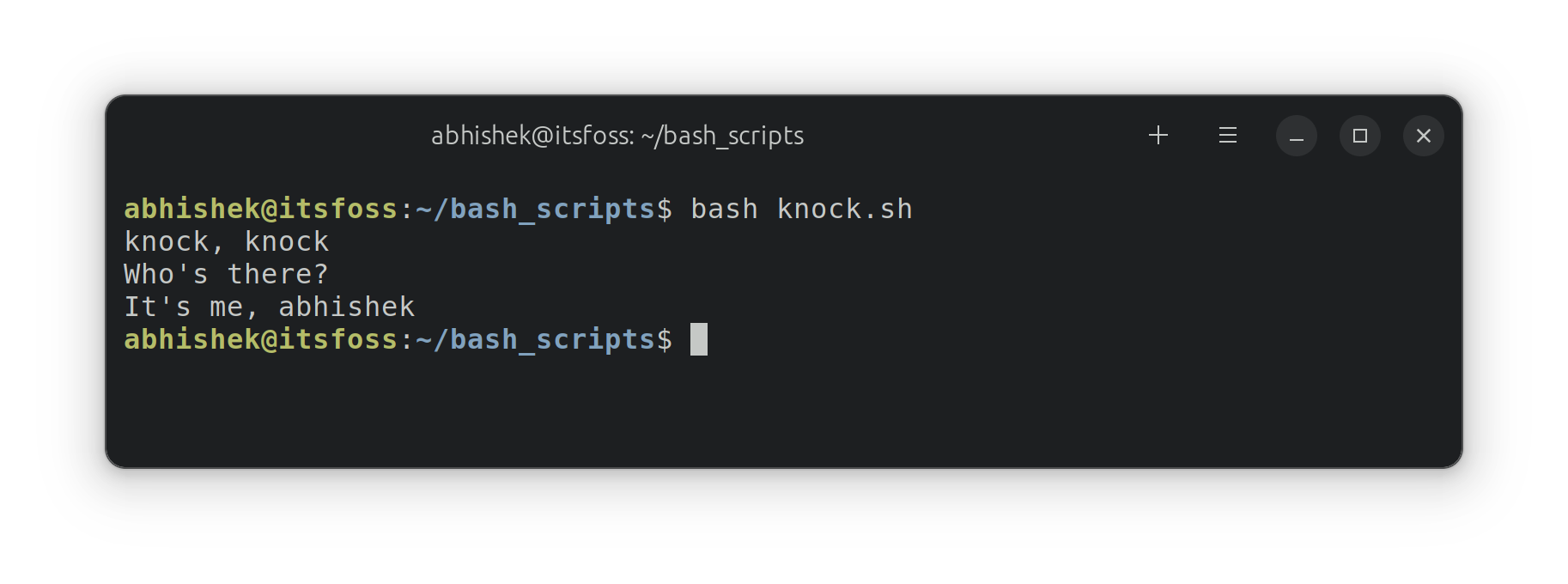
Did you notice how it added my name to it automatically? That’s the magic of the global variable $USER that contains the username.
You may also notice that I used the ” sometimes with echo but not other times. That was deliberate. Quotes in bash have special meanings. They can be used to handle white spaces and other special characters. Let me show an example.
Handling spaces in variables
Let’s say you have to use a variable called greetings
that has the value hello and welcome
.
If you try initializing the variable like this:
greetings=Hello and Welcome
You’ll get an error like this:
Command 'and' not found, but can be installed with:
sudo apt install and
This is why you need to use either single quotes or double quotes:
greetings="Hello and Welcome"
And now you can use this variable as you want.

Assign the command output to a variable
Yes! You can store the output of a command in a variable and use them in your script. It’s called command substitution.
var=$(command)
Here’s an example:
[email protected]:~$ today=$(date +%D)
[email protected]:~$ echo "Today's date is $today"
Today's date is 06/19/23
[email protected]:~$

The older syntax used backticks instead of $() for the command substitution. While it may still work, you should use the new, recommended notation.
💡
Variables change the value unless you declare a ‘constant’ variable like this: readonly pi=3.14
. In this case, the value of variable pi
cannot be changed because it was declared readlonly
.
🏋️ Exercise time
Time to practice what you learned. Here are some exercise to test your learning.
Exercise 1: Write a bash script that prints your username, present working directory, home directory and default shell in the following format.
Hello, there
My name is XYZ
My current location is XYZ
My home directory is XYZ
My default shell is XYZ
Hint: Use global variables $USER, $PWD, $HOME and $SHELL.
Exercise 2: Write a bash script that declares a variable named price
. Use it to get the output in the following format:
Today's price is $X
Tomorrow's price is $Y
Where X is the initial value of the variable price
and it is doubled for tomorrow’s prices.
Hint: Use / to escape the special character $.
The answers to the exercises can be discussed in this dedicated thread in the community.

In the next chapter of the Bash Basics Series, you’ll see how to make the bash scripts interactive by passing arguments and accepting user inputs.