This is the beginning of a new tutorial series on It’s FOSS. In this one, you’ll get familiar with bash scripting.
The series assumes that you are somewhat familiar with the Linux terminal. You don’t have to be a master, but knowing the basics would be good. I advise going through Terminal Basics Series.

Who is this series for?
Anyone who wants to start learning bash shell scripting.
If you are a student with shell scripting as part of your course curriculum, this series is for you.
If you are a regular desktop Linux user, this series will help you understand most shell scripts you come across while exploring various software and fixes. You could also use it to automate some common, repetitive tasks.
By the end of this Bash Basics series, you should be able to write simple to moderate bash scripts.
All the chapters in the series have sample exercises so that you can learn it by doing it.
🚧
You’ll learn bash shell scripting here. While there are other shells with mostly the same syntax, their behavior still differs at a few points. Bash is the most common and universal shell and hence start learning shell scripting with bash.
Your first shell script: Hello World!
Open a terminal. Now create a new directory to save all the scripts you’ll be creating in this series:
mkdir bash_scripts
Now switch to this newly created directory:
cd bash_scripts
Let’s create a new file here:
touch hello_world.sh
Now, edit the file and add echo Hello World
line to it. You can do this with the append mode of the cat command (using >):
[email protected]:~/bash_scripts$ cat > hello_world.sh echo Hello World
^C
I prefer adding new lines while using the cat command for adding text.
Press Ctrl+C or Ctrl+D keys to come out of the append mode of the cat command. Now if you check the contents of the script hellow_world.sh
, you should see just a single line.

The moment of truth has arrived. You have created your first shell script. It’s time to run the shell script.
Do like this:
bash hello_world.sh
The echo command simply displays whatever was provided to it. In this case, the shell script should output Hello World on the screen.

Congratulations! You just successfully ran your first shell script. How cool is that!
Here’s a replay of all the above commands for your reference.
Another way to run your shell scripts
Most of the time, you’ll be running the shell scripts in this manner:
./hello_world.sh
Which will result in an error because the file for you as the script doesn’t have execute permission yet.
bash: ./hello_world.sh: Permission denied
Add execute permission for yourself to the script:
chmod u+x hello-world.sh
And now, you can run it like this:
./hello_world.sh

So, you learned two ways to run a shell script. It’s time to focus on bash.
Turn your shell script into a bash script
Confused? Actually, there are several shells available in Linux. Bash, ksh, csh, zsh and many more. Out of all these, bash is the most popular one and almost all distributions have it installed by default.
The shell is an interpreter. It accepts and runs Linux commands. While the syntax for most shell remains the same, their behavior may differ at certain points. For example, the handling of brackets in conditional logic.
This is why it is important to tell the system which shell to use to interpret the script.
When you used bash hello_world.sh
, you explicitly used the bash interpreter.
But when you run the shell scripts in this manner:
./hello_world.sh
The system will use whichever shell you are currently using to run the script.
To avoid unwanted surprises due to different syntax handling, you should explicitly tell the system which shell script it is.
How to do that? Use the shebang (#!). Normally, # is used for comments in shell scripts. However, if #! is used as the first line of the program, it has the special purpose of telling the system which shell to use.
So, change the content of the hello_world.sh so that it looks like this:
#!/bin/bash echo Hello World
And now, you can run the shell script as usual knowing that the system will use bash shell to run the script.
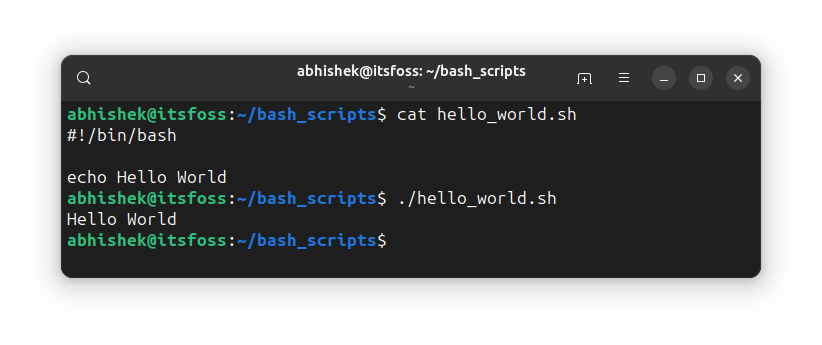
💡
If you feel uncomfortable editing script files in the terminal, as a desktop Linux user, you can use Gedit or other GUI text editors for writing scripts and run them in the terminal.
🏋️ Exercise time
It is time to practice what you learned. Here are some basic practice exercises for this level:
- Write a bash script that prints “Hello Everyone”
- Write a bash script that displays your current working directory (hint: use pwd command)
- Write a shell script that prints your user name in the following manner: My name is XYZ (hint: use $USER)
The answers can be discussed in this dedicated thread in the Community forum.

The last practice exercise uses $USER
. That’s a special variable that prints the user name.
And that brings me to the topic of the next chapter in the Bash Basics Series: Variables.
Stay tuned for that next week.